Yowatech.id – Script Python Gambar adalah sebuah kode yang menggunakan bahasa pemrograman Python dan library turtle untuk membuat gambar sederhana seperti persegi, lingkaran, atau pola lainnya.
Baca Juga: 5+ Contoh Program Python if else
Turtle library membuat tampilan visual dari kode dan memudahkan pemrogram untuk membuat gambar menggunakan bahasa pemrograman Python. Program ini dapat mengatur posisi dan arah dari turtle untuk membuat garis atau membuat lingkaran dan membuat gambar yang lebih kompleks.
Cara Menjalankan Turtle library:
Untuk menjalankan Turtle library Python, Anda bisa menggunakan beberapa cara berikut:
- Menjalankan di IDLE Python: Buka IDLE Python, buat file baru, masukkan kode yang menggunakan Turtle library, dan jalankan file tersebut dengan menekan F5 atau dengan memilih Run -> Run Module dari menu.
- Menjalankan di terminal atau command prompt: Buka terminal atau command prompt, arahkan ke direktori yang menyimpan file Python, dan jalankan file tersebut dengan mengetikkan “python nama_file.py”.
- Menjalankan di Jupyter Notebook: Buka Jupyter Notebook, buat sel baru, masukkan kode yang menggunakan Turtle library, dan jalankan sel tersebut dengan menekan Shift + Enter.
Pastikan bahwa Anda sudah menginstal Turtle library sebelum menjalankan program yang menggunakannya. Anda bisa menginstalnya dengan mengetikkan “pip install turtle” pada terminal atau command prompt.
Baca Juga: 6+ Contoh Program Python Perpustakaan
Contoh 1 Script Python Gambar Persegi
Berikut adalah contoh script Python untuk membuat gambar persegi menggunakan turtle library:
import turtle
def draw_square(t, size):
for i in range(4):
t.forward(size)
t.right(90)
t = turtle.Turtle()
t.speed(0)
draw_square(t, 100)
turtle.done()
Output:
Contoh 2 Script Python Gambar Turtle
Berikut adalah contoh script Python untuk membuat gambar kura-kura menggunakan turtle library:
import turtle
from helpercode import checkpos, intersect, Counter
import random
screen = turtle.Screen()
screen.setworldcoordinates(-400,-400,400,400)
class Runner(turtle.Turtle):
def __init__(self):
turtle.Turtle.__init__(self)
self.shape('turtle')
self.penup()
screen.tracer(10)
self.setpos(-100,0)
self.seth(0)
self.pendown()
screen.tracer(1)
def reset(self):
self.hideturtle()
self.clear()
self.__init__()
# A list of all of our chasers
chasers = []
class Chaser(turtle.Turtle):
def __init__(self, target, coordinates = [100,0]):
turtle.Turtle.__init__(self)
self.target = target
self.start = coordinates
self.shape('turtle')
c1 = abs(coordinates[0])
c2 = abs(coordinates[1])
c3 = max(255, abs(sum(coordinates)))
self.color(c1, c2, c3)
self.penup()
screen.tracer(0)
self.setpos(self.start)
self.seth(self.towards(target))
self.pendown()
screen.tracer(1)
# Add Self to list of Chasers
chasers.append(self)
def reset(self):
self.hideturtle()
self.clear()
self.__init__(self.target)
def track(self, move=False):
screen.tracer(0)
self.seth(self.towards(self.target) + random.randint(-25,25))
self.width(self.distance(self.target.pos()) //20)
if intersect(self.target,self):
x,y = self.pos()
c1 = abs(x)
c2 = abs(y)
c3 = max(256, abs(sum([x,y])))
self.color((c1, c2, c3))
self.penup()
self.goto(self.start)
self.pendown()
elif move:
self.forward(9+random.randint(-4,0))
else:
self.forward(1)
checkpos([self.target,self],screen)
screen.tracer(1)
tina = Runner()
tina.write("Click and Drag!")
tina.backward(20)
tommy = Chaser(tina, [200,-50])
sally = Chaser(tina, [50,200])
johnny = Chaser(tina, [-150,-100])
# Reset the game to original state
def reset():
tina.reset()
tommy.reset()
# Define functions for each arrow key
def go_left():
screen.tracer(0)
tina.left(7)
tommy.track()
def go_right():
screen.tracer(0)
tina.right(7)
tommy.track()
def go_forward():
screen.tracer(0)
tina.forward(10)
tommy.track(move=True)
def go_backward():
screen.tracer(0)
tina.backward(10)
tommy.track(move=True)
# Tell the program which functions go with which keys
screen.onkey(go_left, 'Left')
screen.onkey(go_right, 'Right')
screen.onkey(go_forward, 'Up')
screen.onkey(go_backward, 'Down')
def drag_function(x,y):
screen.tracer(0)
tina.clear()
tina.goto(x,y)
for chaser in chasers:
chaser.track(True)
screen.tracer(1)
tina.ondrag(drag_function)
# Reset the game when the user presses 'r'
screen.onkey(reset,"r")
# Tell the screen to listen for key presses
screen.listen()
turtle.done()
Helper Code:
import turtle
counterscreen = turtle.Screen()
counterscreen.bgcolor("palegoldenrod")
def checkpos(turtlelist, screen):
"""
Takes a list of turtle objects and a Screen object.
Sets the turtles to the opposite edge of the screen.
"""
screen.tracer(0)
for turtle in turtlelist:
dim = 400
turtle.penup()
x = turtle.xcor()
y = turtle.ycor()
if x > dim:
turtle.setx(-1 * dim)
elif x < -1 * dim:
turtle.setx(dim)
if y > dim:
turtle.sety(-1* dim)
elif y < -1 * dim:
turtle.sety(dim)
turtle.pendown()
screen.tracer(1)
return
def intersect(object1, object2, radius = 15):
"""
Takes two Turtle objects and an optional radius.
Returns True if the objects are less than radius apart.
"""
xbounds = (object1.xcor()-radius, object1.xcor() + radius)
ybounds = (object1.ycor()-radius, object1.ycor() + radius)
x, y = object2.pos()
check_x = x > min(xbounds) and x < max(xbounds)
check_y = y > min(ybounds) and y < max(ybounds)
if (check_x and check_y):
return True
else:
return False
class Counter(turtle.Turtle):
def __init__(self, coordinates = [160, 170], screen = counterscreen):
turtle.Turtle.__init__(self)
self.count = 0
self.hideturtle()
self.speed(0)
self.penup()
self.goto(coordinates)
self.screen = screen
def show(self, message, alignment = "right", size = 18):
self.screen.tracer(0)
self.clear()
self.write(message,font=("Arial",size),align=alignment)
self.screen.tracer(1)
def increment(self):
self.count += 1
def showcount(self, x = 0, y = 0):
self.increment()
self.show(self.count)

Output:
Contoh 3 Script Python Gambar Bunga
Berikut adalah contoh script Python untuk membuat gambar bunga warna-warni menggunakan turtle library:
import turtle
import math
import random
wn = turtle.Screen()
wn.bgcolor('black')
Albert = turtle.Turtle()
Albert.speed(0)
Albert.color('white')
rotate=int(360)
def drawCircles(t,size):
for i in range(10):
t.circle(size)
size=size-4
def drawSpecial(t,size,repeat):
for i in range (repeat):
drawCircles(t,size)
t.right(360/repeat)
drawSpecial(Albert,100,10)
Steve = turtle.Turtle()
Steve.speed(0)
Steve.color('yellow')
rotate=int(90)
def drawCircles(t,size):
for i in range(4):
t.circle(size)
size=size-10
def drawSpecial(t,size,repeat):
for i in range (repeat):
drawCircles(t,size)
t.right(360/repeat)
drawSpecial(Steve,100,10)
Barry = turtle.Turtle()
Barry.speed(0)
Barry.color('blue')
rotate=int(80)
def drawCircles(t,size):
for i in range(4):
t.circle(size)
size=size-5
def drawSpecial(t,size,repeat):
for i in range (repeat):
drawCircles(t,size)
t.right(360/repeat)
drawSpecial(Barry,100,10)
Terry = turtle.Turtle()
Terry.speed(0)
Terry.color('orange')
rotate=int(90)
def drawCircles(t,size):
for i in range(4):
t.circle(size)
size=size-19
def drawSpecial(t,size,repeat):
for i in range (repeat):
drawCircles(t,size)
t.right(360/repeat)
drawSpecial(Terry,100,10)
Will = turtle.Turtle()
Will.speed(0)
Will.color('pink')
rotate=int(90)
def drawCircles(t,size):
for i in range(4):
t.circle(size)
size=size-20
def drawSpecial(t,size,repeat):
for i in range (repeat):
drawCircles(t,size)
t.right(360/repeat)
drawSpecial(Will,100,10)
Output:
Contoh 4 Script Python Gambar Rumah
Berikut adalah contoh script Python untuk membuat gambar rumah warna-warni menggunakan turtle library:
import turtle
wn = turtle.Screen()
bird = turtle.Turtle()
bird.speed(10)
bird.pensize(4)
bird.color("OliveDrab")
bird.setpos(-50, 0)
bird.fillcolor("Olive")
bird.begin_fill()
for i in [0, 1, 2]:
bird.forward(175)
bird.left(120)
bird.end_fill()
bird.forward(25)
bird.right(90)
bird.fillcolor("PeachPuff")
bird.begin_fill()
for i in [0, 1, 2]:
bird.fd(125)
bird.left(90)
bird.end_fill()
bird.penup()
bird.fd(62.5)
bird.left(90)
bird.forward(25)
bird.right(90)
bird.pendown()
bird.color("Black")
bird.fillcolor("Black")
bird.begin_fill()
bird.circle(15,360)
bird.end_fill()
bird.hideturtle()
wn.exitonclick()
Output:
Contoh 5 Script Python Gambar Salju
# Geek Gurl Diaries Episode 33: Xmas Special Make Snowflakes with Turtle
# By Carrie Anne Philbin
# https://www.youtube.com/watch?v=DHmeX7YTHBY
import turtle
import random
# setup the window with a background colour
wn = turtle.Screen()
wn.bgcolor("cyan")
# assign a name to your turtle
elsa = turtle.Turtle()
elsa.speed(0)
# create a list of colours
sfcolor = ["white", "blue", "purple", "grey", "magenta"]
# create a function to create different size snowflakes
def snowflake(size):
# move the pen into starting position
elsa.penup()
elsa.forward(10*size)
elsa.left(45)
elsa.pendown()
elsa.color(random.choice(sfcolor))
# draw branch 8 times to make a snowflake
for i in range(8):
branch(size)
elsa.left(45)
# create one branch of the snowflake
def branch(size):
for i in range(3):
for i in range(3):
elsa.forward(10.0*size/3)
elsa.backward(10.0*size/3)
elsa.right(45)
elsa.left(90)
elsa.backward(10.0*size/3)
elsa.left(45)
elsa.right(90)
elsa.forward(10.0*size)
# loop to create 20 different sized snowflakes with different starting co-ordinates
for i in range(20):
x = random.randint(-200, 200)
y = random.randint(-200, 200)
sf_size = random.randint(1, 4)
elsa.penup()
elsa.goto(x, y)
elsa.pendown()
snowflake(sf_size)
# leave the window open until you click to close
wn.exitonclick()
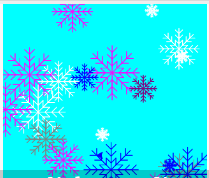
Penutup:
Script Python Turtle adalah bahasa pemrograman yang digunakan untuk membuat gambar dan animasi sederhana. Menggunakan Turtle, Anda dapat membuat gambar yang dibentuk oleh garis dan lingkaran dengan memanfaatkan perintah yang tersedia. Ini sangat berguna untuk memvisualisasikan konsep matematika dan membantu memahami konsep seperti pengaturan, rotasi, dan skala.
Script Python Turtle juga merupakan cara yang bagus untuk mempelajari dasar-dasar pemrograman karena memiliki antarmuka yang sederhana dan mudah dipahami. Ini memungkinkan Anda untuk fokus pada logika dan alur program sambil membangun kemampuan pemrograman yang lebih besar.
Baca Juga: 6+ Contoh Program Python Perpustakaan